Unix Socket Programming (Contd..)
Sequence of System Calls for Connectionless Communication
The typical set of system calls on both the machines in a connectionless setup is shown in Figure below.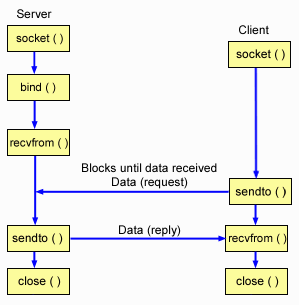
- The socket and bind system calls are called in the same way as in the connection-oriented case. Again the bind call is optional at the client side.
- The connect function is not called in a connectionless communication with the sane intention as above. Instead, if we call a connect() in this case, then we are simply specifying a particular server address to which we have to send, and from which we have to receive the Datagrams
- Every time a packet has to be sent over a socket, the remote address has to be mentioned. This is because there is no concept of a connection that can remember which remote machine to send that packet to.
- The calls sendto and recvfrom are used to send
datagram packets. The synopses of both are given below. int sendto(int
skfd, void *buf, int buflen, int flags, struct sockaddr* to, int tolen);
int recvfrom(int skfd, void *buf, int buflen, int flags, struct
sockaddr* from, int fromlen);
sendto sends a datagram packet containing the data present in buf addressed to the address present in the sockaddr structure, to.
recvfrom fills in the buf structure with the data received from a datagram packet and the sockaddr structure, from with the address of the client from which the packet was received.
Both these calls block until a packet is sent in case of sendto and a packet is received in case of recvfrom. In the strict sense though sendto is not blocking as the packet is sent out in most cases and sendto returns immediately. - Suppose if the program desires to communicate only to one particular machine and make the operating system discard packets from all other machines, it can use the connect call to specify the address of the machine with which it will exclusively communicate. All subsequent calls do not require the address field to be given. It will be understood that the remote address is the one specified in connect called earlier.
Socket Options and Settings
There are various options which can be set for a socket and there are multiple ways to set options that affect a socket.Of these, setsockopt() system call is the one specifically designed for this purpose. Also, we can retrieve the option which are currently set for a socket by means of getsockopt() system call.
int setsockopt(int socket, int level, int option_name, const void *option_value, socklen_t option_len);
The socket argument must refer to an open socket descriptor. The level specifies who in the system is to interpret the option: the general socket code, the TCP/IP code, or the XNS code. This function sets the option specified by the option_name, at the protocol level specified by the level, to the value pointed to by the option_value for the socket associated with the file descriptor specified by the socket. The level argument specifies the protocol level at which the option resides. To set options at the socket level, we need to specify the level argument as SOL_SOCKET. To set options at other levels, we need to supply the appropriate protocol number for the protocol controlling the option. The option_name specifies a single option to set. The option_name and any specified options are passed uninterpreted to the appropriate protocol module for interpretations. The list of options available at the socket level (SOL_SOCKET) are:
- SO_DEBUG
- SO_BROADCAST
- SO_REUSEADDR
- SO_KEEPALIVE
- SO_LINGER
- SO_OOBINLINE
- SO_SNDBUF
- SO_RCVBUF
- SO_DONTROUTE
- SO_RCVLOWAT
- SO_RCVTIMEO
- SO_SNDLOWAT
- SO_SNDTIMEO
For boolean options, 0 indicates that the option is disabled and 1 indicates that the option is enabled.Options at other protocol levels vary in format and name.
Some of the options available for the IP_PROTO_TCP socket are:
- TCP_MAXSEG
- TCP_NODELAY
int getsockopt(int socket, int level, int option_name, void *option_value, socklen_t *option_len);
This function retrieves the value for the option specified by the option_name argument for the socket. If the size of the option value is greater than option_len, the value stored in the object pointed to by the option_value will be silently truncated. Otherwise, the object pointed to by the option_len will be modified to indicate the actual length of the value. The level specifies the protocol level at which the option resides. To retrieve options at the socket level, we need to specify the level argument as SOL_SOCKET. To retrieve options at other levels, we need to supply the appropriate protocol number for the protocol controlling the option. The socket in use may require the process to have appropriate privileges to use the getsockopt() function. The list of options for option_name is the same as those available for setsockopt() system call.
Servers
Normally a client process is started on the same system or on another system that is connected to the server's system with a network. Client processes are often initiated by the interactive user entering a command to a time-sharing system. The client process sends a request across the network to the server requesting service of some form. In this way, normally a server handles only one client at a time. If multiple client connections arrive at about the same time, the kernel queues them upto some limit, and returns them to accept function one at a time. But if the server takes more time to service each client (say a few seconds or minutes), we would need some way to overlap the service of one client with another client. Multiple requests can be handled in two ways. In fact servers are classified on the basis of the way they handle multiple requests.- Iterative Servers: When a client's request can be handled by the server in a known, finite amount of time, the server process handles the request itself. These servers handles one client at a time by iterating between them.
- Concurrent Servers: These servers handle multiple clients at the same time. Among the various approaches that are available to handle these multiple clients, simplest approach is to call the UNIX fork system call , creating a child process for each client. When a connection is established, accept returns, the server calls fork, and then the child process services the client and the parent process waits for another connection. The parent closes the connected socket since the child handles this new client.
Internet Superserver (inetd)
Servers must keep running at all times. However, all the servers are not working all this time but merely waiting for a request from a client. To avoid this waste of resources, a single server is run which waits on all the port numbers. This Internet super server is called inetd in UNIX. It is referred to as the ``Internet Super-Server'' because it manages connections for several daemons. Programs that provide network service are commonly known as daemons. inetd serves as a managing server for other daemons. When a connection is received by inetd, it determines which daemon the connection is destined for, spawns the particular daemon and delegates the socket to it. Running one instance of inetd reduces the overall system load as compared to running each daemon individually in stand-alone mode. This daemon provides two features -1. It allows a single process (inetd) to be waiting to service multiple connection requests, instead of one process for each potential service. This reduces the total number of processes in the system.
2. It simplifies the writing of the server processes to handle the requests, since many of the start-up details are handled by inetd.
The inetd process uses fork and exec system calls to invoke the actual server process.The only way the server can obtain the identity of the client is by calling the getpeername system call.
int getpeername(int sockfd, struct sockaddr *peer, int *addrlen);
Getpeername returns the name of the peer connected to socket sockfd. The addrlen parameter should be initialised to indicate the amount of space pointed to by peer . On return it contains the actual size of the name returned (in bytes). The name is truncated if the buffer provided is too small.
A similar system call is getsockname. Getsockname returns the current name for the specified socket. The addrlen parameter should be initialized to indicate the amount of space pointed to by name. On return it contains the actual size of the name returned (in bytes).
int getsockname(int sockfd, struct sockaddr *name, int *addrlen);
Following illustrates the steps performed by inetd
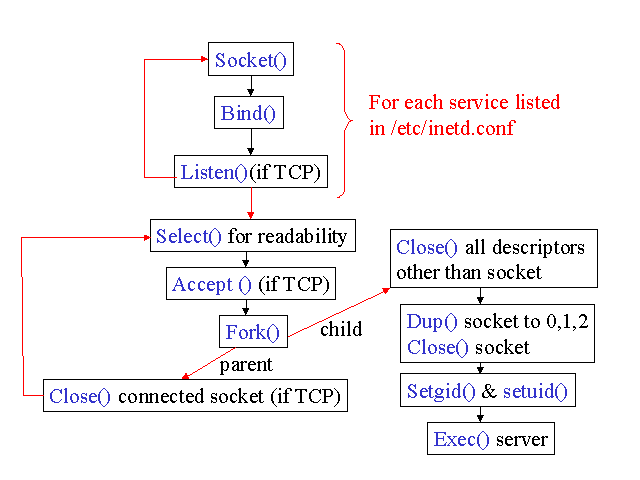
Image References
- http://publib.boulder.ibm.com/iseries/v5r1/ic2924/info/rzab6/rxab6503.gif
- http://www.cs.odu.edu/~cs779/spring03/lectures/inetd.gif
0 comments:
Post a Comment